Integrate with ConnectWallet
If you also want to integrate this wallet with ConnectWallet
component in React SDK or React Native SDK, You have to create a Wallet Configurator function which can be passed to ThirdwebProvider
component
Required
Optional
Creating wallet connection UI
Connecting your wallet in wallet connection UI
There are two ways to connect your wallet in connectUI
Rendering a custom UI for selecting your wallet
Instead of the default icon + name in the wallet selector screen, you can render a custom UI for your wallet.
Take Embedded wallet for example, It renders Social Icons + Email Input.
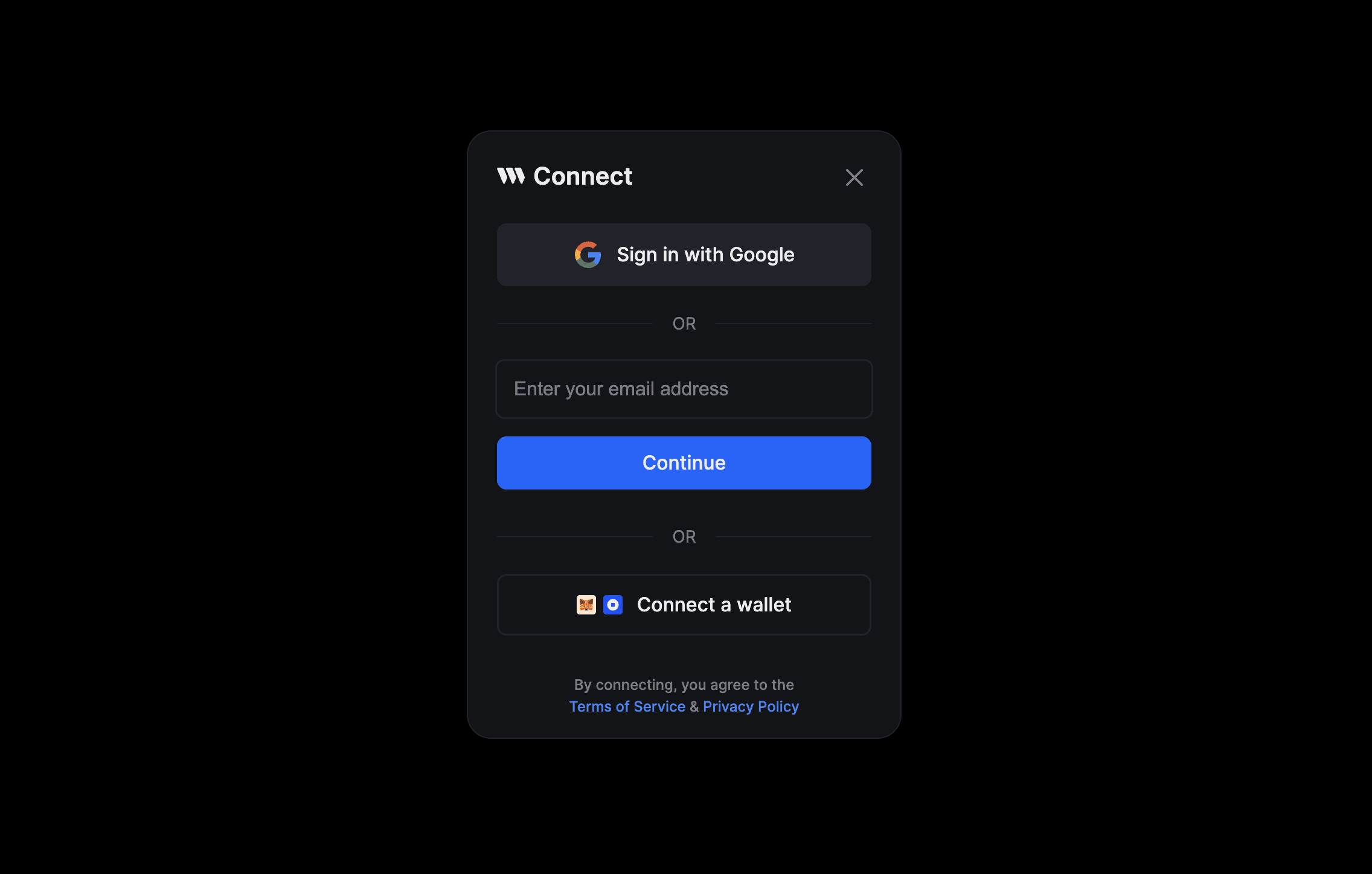
This can be done by specifying a React component in the selectUI
property of your wallet config.
Add your wallet to ThirdwebProvider
You can now use your wallet with the Connect Wallet button! Simply add it to the supportedWallets
prop of the ThirdwebProvider
.
Examples
You can look at how thirdweb-dev/react
package's built-in wallets are implemented for reference:
Contributing to the Wallets package
If you think your wallet implementation would be useful to others, please consider sharing it by opening a PR to the @thirdweb-dev/wallets
package.